XYLEM AND PHLOEM NUMBER IN JAVA LANGUAGE
AMRUHA AHMED
6th October,2022.
A Xylem Number is the number whose sum of extreme digits is equal to the sum of mean digits. A Phloem Number is the number whose sum of extreme digits is not equal to the sum of mean digits
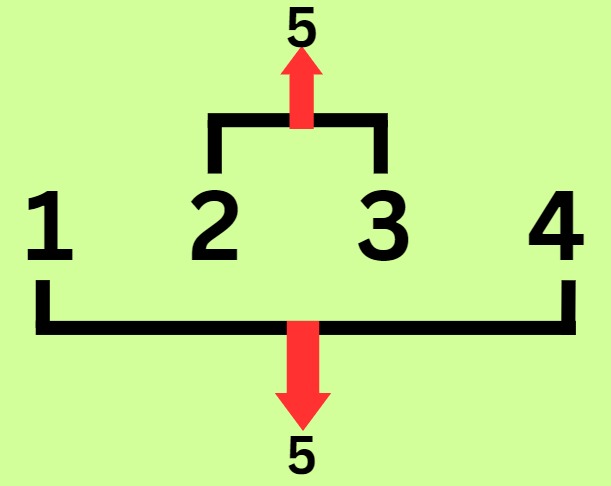
The following Java program checks whether the given number is a Xylem Number or a Phloem Number:
METHOD-1:
import java.util.*;
class XylemandPhloemNumber
{
public static void main(String []args)
{
Scanner ob=new Scanner(System.in);
int n;//accepted number
int a[]=new int[20];//array to store digits of n
int i=0;//index of array a[]
int ctr;//count of digits
int j;//loop counter
int s1=0,s2=0;// s1- sum of extreme digits,s2=sum of digits in middle
int t;//dummy variable
System.out.print("Enter a number:");
n=ob.nextInt();
t=n;
while(n!=0)
{
int rem=n%10;
a[i++]=rem;
n=n/10;
}
ctr=i;
s1=a[ctr-1]+a[0];
for(j=1;j<ctr-1;j++)
s2=s2+a[j];
if(s1==s2)
System.out.println(t+" is a Xylem Number");
else
System.out.println(t+" is a Phloem Number");
}
}
DRY RUN:
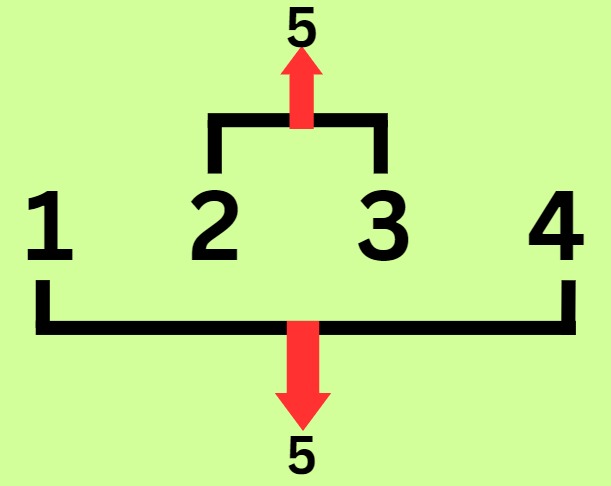
Considering n as 1234. From the above image, it can be safely said that n is a Xylem Number
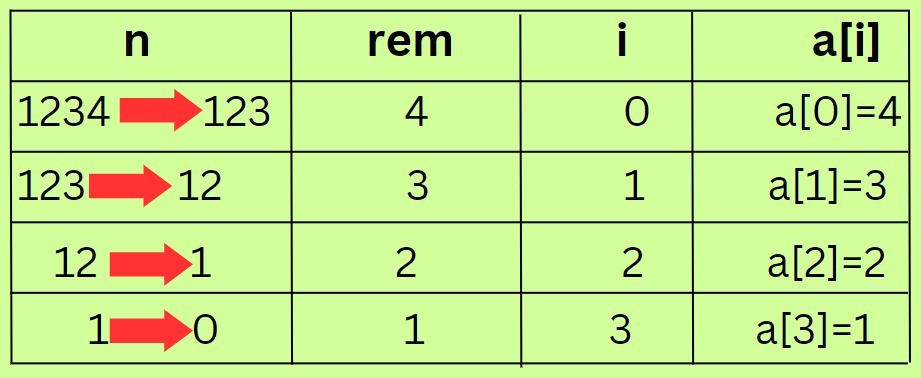
Extracting all the digits of the number n using a while loop and storing it in array a[] using the loop counter i as shown

The array a[] contains digits of the number n in a reversed fashion.
NOTE: The order of arrangement of digits in the array does not
affect the final result. In case of any confusion arising, a suitable loop can be used to reverse the order of storage of digits .
Now, ctr is assigned the value of i and the sum of extreme digits s1 is calculated. In this case, s1 is equal to 5.
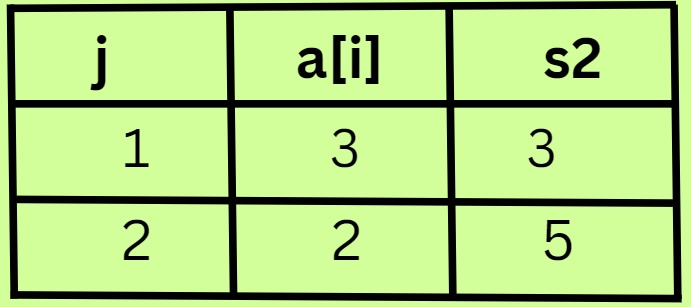
Using a for loop of loop counter j, the sum of mean digits or s2 is calculated.
At the end of the for loop, s2 is calculated as 5.
Now, s1 and s2 are checked for equality. In this case, both s1 and s2 are equal to 5. Hence the condition for Xylem Number is satisfied.
OUTPUT:
enter a number:1234
1234 is a Xylem Number
DRY RUN FOR PHLOEM NUMBER:
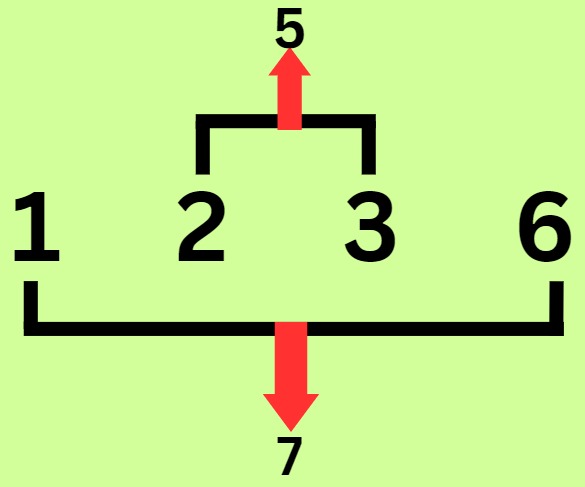
Considering n as 1236. From the above image, it can be safely said that n is a Phloem Number
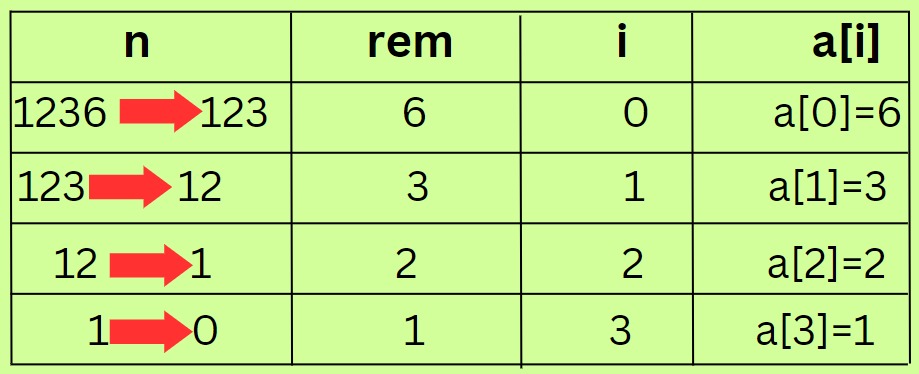
Extracting all the digits of the number n using a while loop and storing it in array a[] using the loop counter i as shown
s
The array a[] contains digits of the number n in a reversed fashion.
NOTE: The order of arrangement of digits in the array does not
affect the final result. In case of any confusion arising, a suitable loop can be used to reverse the order of storage of digits .
Now, ctr is assigned the value of i and the sum of extreme digits s1 is calculated. In this case, s1 is equal to 7.
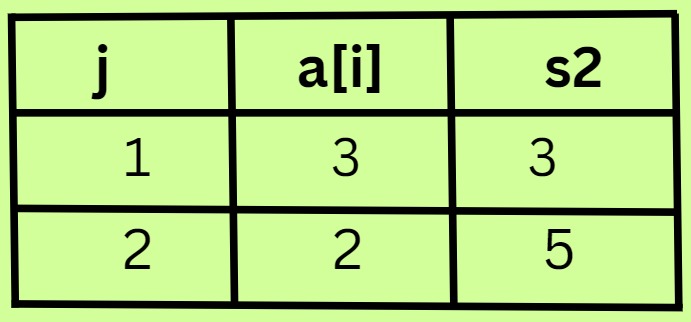
Using a for loop of loop counter j, the sum of mean digits or s2 is calculated.
At the end of the for loop, s2 is calculated as 5.
Now, s1 and s2 are checked for equality. In this case, both s1 and s2 are not equal to each other. Hence the condition for Xylem Number is not satisfied anfd the number n is designated as a Phloem Number
OUTPUT:
enter a number:1236
1234 is a Phloem Number
METHOD-2:
import java.util.*;
class XylemandPhloemNumber{
public static void main(String []args)
{
Scanner ob=new Scanner(System.in);
int n;//accepted number
int s1=0;//sum of extreme digits
int s2=0;//sum of middle digits
int d;//dummy variable
System.out.print("Enter a number:");
n=ob.nextInt();
d=n;
s1=n%10;
n=n/10;
while(n>9)
{
s2=s2+n%10;
n=n/10;
}
s1=s1+n;
if(s1==s2)
System.out.println(d+" is a Xylem Number");
else
System.out.println(d+" is a Phloem Number");
}
}
DRY RUN FOR XYLEM NUMBER:
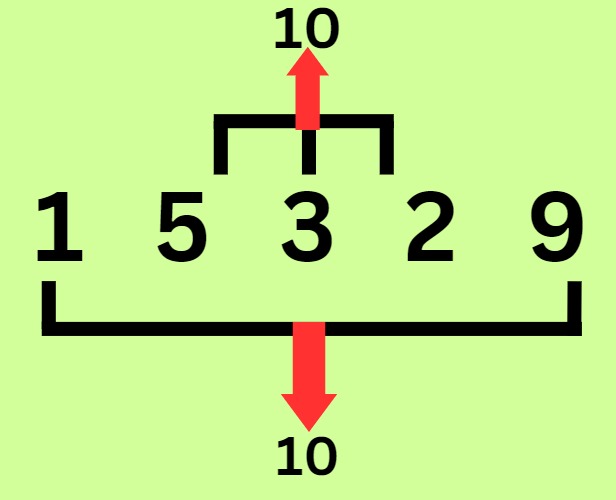
Considering n as 15329. From the above image, it can be safely said that n is a Xylem Number
Here, s1 is first calculated as the n%10 in order to extract the last digit of the number
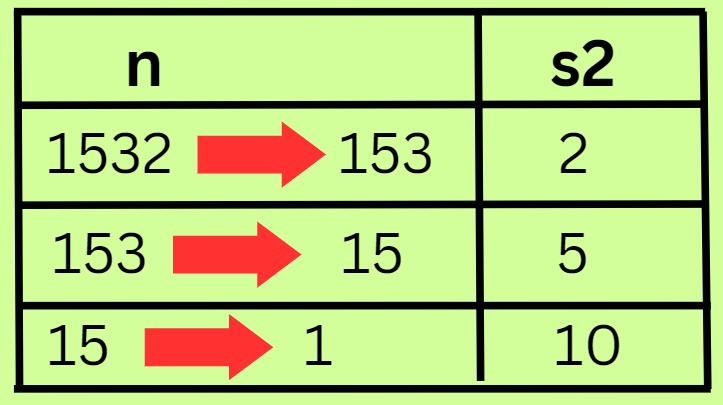
Using the while loop, the sum of mean digits is calculated and stored in s2. This loop stops when n is reduced to a single digit number.
Now, s1 is calculated at s1+=n in order to extract the first digit of 15329.Since s1 and s2 are equal to 10 hence 15329 is designated as a Xylem Number.
OUTPUT:
enter a number:15329
15329 is a Xylem Number
DRY RUN FOR PHLOEM NUMBER:
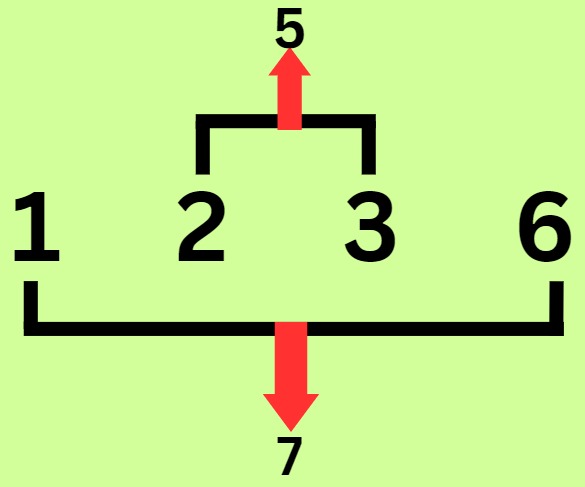
Considering n as 1326. From the above image, it can be safely said that n is a Phloem Number
Here, s1 is first calculated as the n%10 in order to extract the last digit of the number
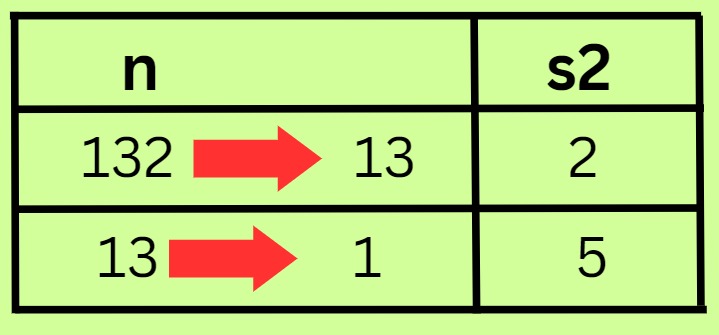
Using the while loop, the sum of mean digits is calculated and stored in s2. This loop stops when n is reduced to a single digit number.
Now, s1 is calculated at s1+=n in order to extract the first digit of 15329.Since s1 is equal to 7 and s2 is equal to 5 hence 1326 is designated as a Phloem Number.
OUTPUT:
enter a number:1326
1326 is a Phloem Number