SPY NUMBER IN PYTHON LANGUAGE
AMRUHA AHMED
12th May,2023.
A number whose sum of digits is equal to the product of digits is said to be a Spy Number.
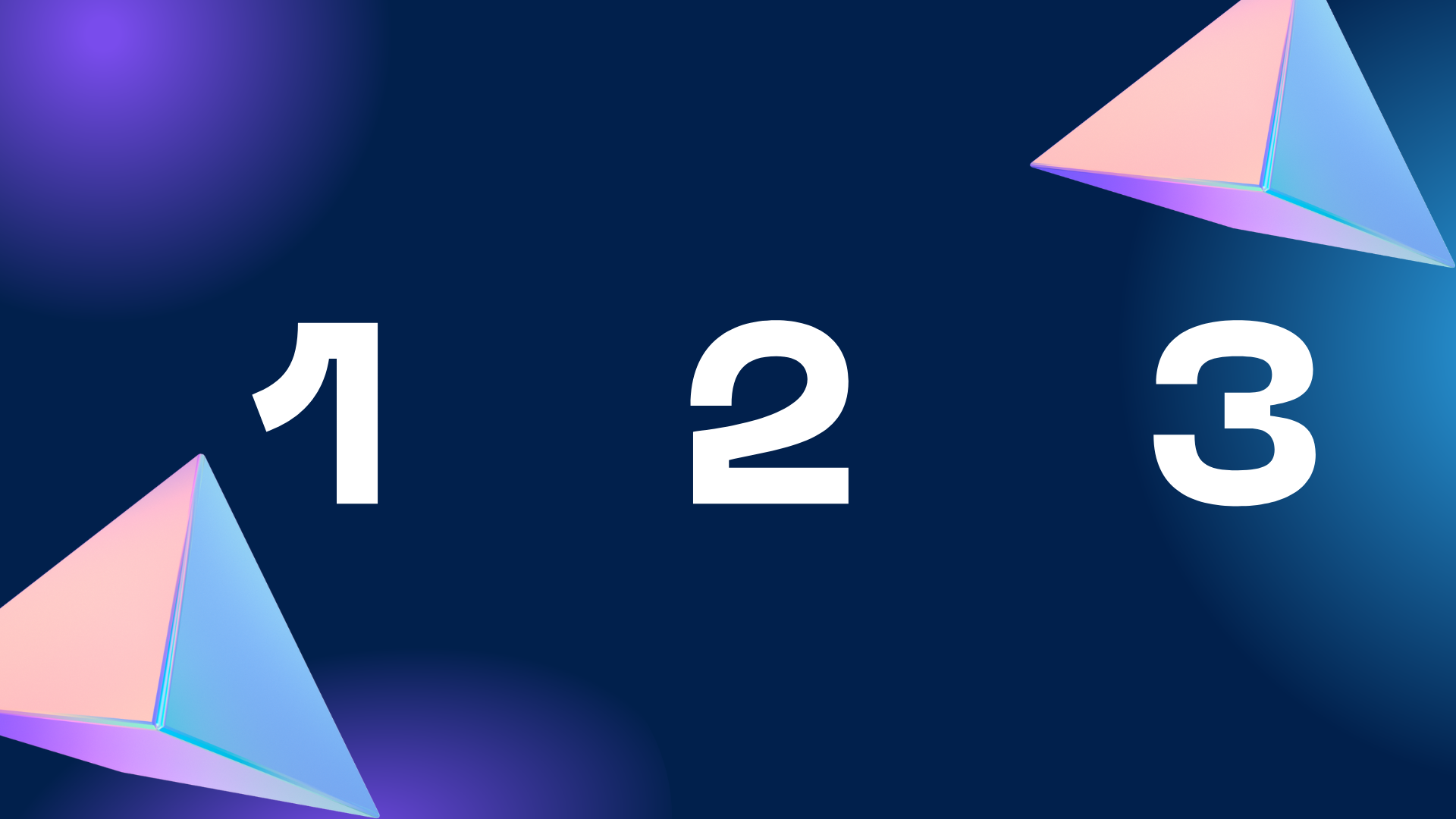
DELVE DEEPER:
In order to check whether the given number is a spy number or not, we require two functions :
- sum_of_digits() - to calculate the sum of digits of the number
- product_of_digits () -to calculate the product of digits of the number
ALGORITHM FOR SUM_OF_DIGITS FUNCTION:
- 1.start
- 2. The number "n" is passed as parameter to this function
- 3.Variable s is initialized to 0 for storing the sum of digits of "n".
- 4.while "n" is not equal to 0, the following steps need to be repeated:
- In “rem” store the last digit of n
- Calculate s as s+=rem
- Update n as n//=10
- 5.Stop
ALGORITHM FOR PRODUCT_OF_DIGITS FUNCTION:
- 1.start
- 2. The number "n" is passed as parameter to this function
- 3.Variable p is initialized to 1 for storing the product of digits of "n".
- while "n" is not equal to 0, the following steps need to be repeated:
- In “rem” store the last digit of n
- Calculate p as p=p*rem
- Update n as n//=10
- 5.Stop
The following Python program checks whether the given number is a Spy Number or not:
PROGRAM:
def sum_of_digits(n):
s=0 #sum
while n!=0 :
rem=n%10 #extracting the last digit of n
s=s+rem #calculating sum
n=n//10 #updating n
return s
def product_of_digits(n):
p=1 #product
while n!=0:
rem=n%10 #extracting the last digit of n
p=p*rem #calculating product
n=n//10 #updating n
return p
n=int(input("Enter a number:")) #n-number
sum=sum_of_digits(n) #storing value of sum_of_digits() in sum
product=product_of_digits(n) #storing value of product_of_digits() in product
if(sum==product): #checking condition for spy number
print("{} is a Spy Number".format(n))
else:
print("{} is not a Spy Number".format(n))
DRY RUN:
Suppose n=123 is passed as parameter to the sum function . The values of s,rem and n will be:
If we pass n=123 as parameter to the product function, the values of n,rem and p will be:
Since value returned from sum function is 6 and the value returned from product function is also 6, hence the number 123 is a spy number.
OUTPUT:
Enter a number:123
123 is a Spy Number
OUTPUT:
Enter a number:112
112 is not a Spy Number