GENERATING PRIME PADOVAN SEQUENCE USING C++
AMRUHA AHMED
8th May,2023.
The Padovan Sequence is a special type of sequence in which the n’th term is determined by the recurrence relation;
P(n)=P(n-2)+P(n-3)
Where P(0),P(1) and P(2) are initialized to 1.
The following figure, in which the length of the current triangle's side
is determined by the sum of the lengths of the sides of those triangles
that are touching it, can also be used to understand the Padovan Sequence (assuming that this spiral of equilateral triangle is drawn from inside out).
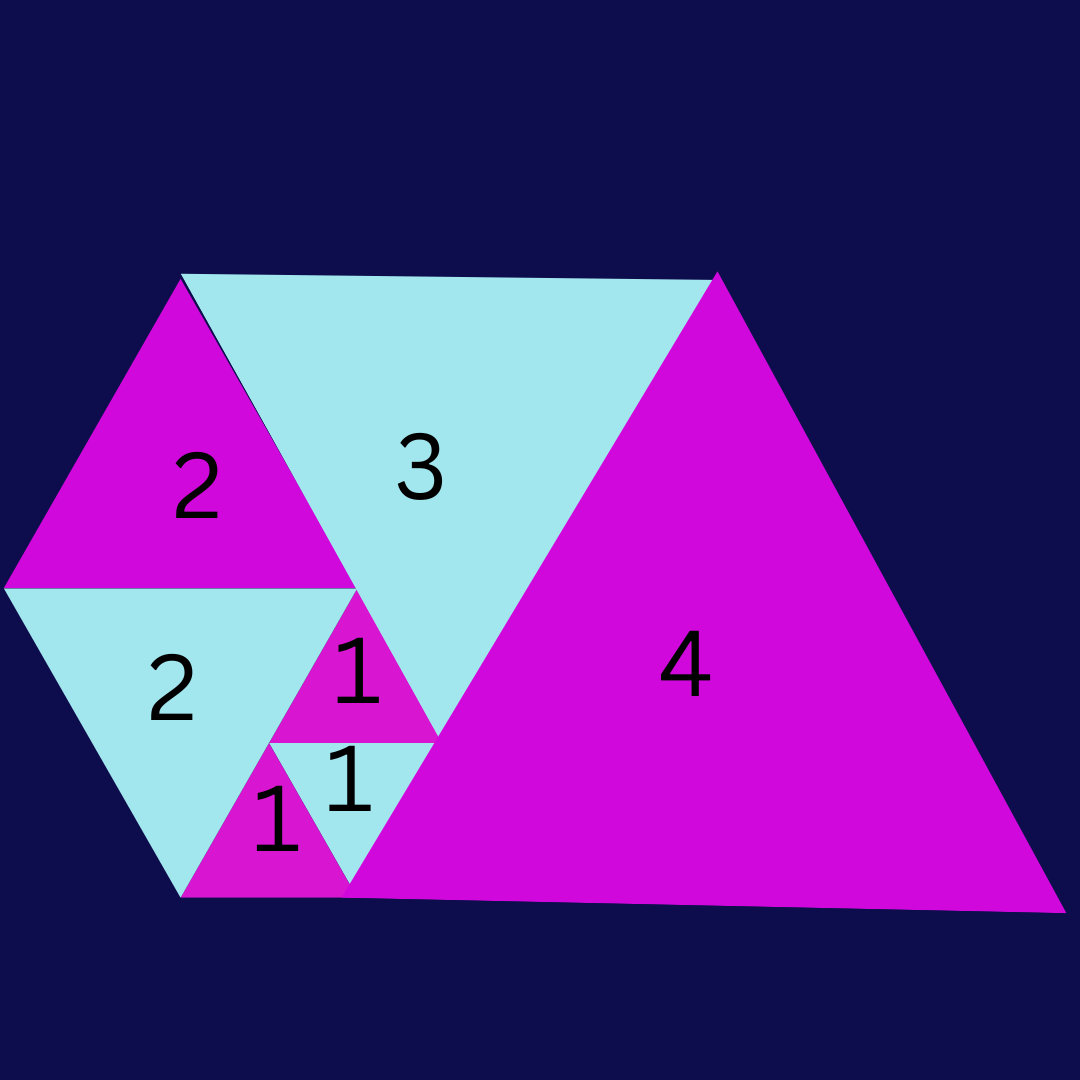
The task at hand is to generate prime padovan sequence till a number; to display only those terms occurring before the limit which are prime as well as a part of the padovan sequence.
VARIABLES REQUIRED:
- n-to store the limit till which the terms need to be printed
- p0 - to store the 0’th term of the sequence
- p1 -to store the 1st term of the sequence
- p2- to store the 2nd term of the sequence
- pn-to store the n’th term of the sequence
- i-loop counter for checking whether pn is prime or not.
- ctr-counter to store the number of factors of pn.
- 1.Accept the limit till which the series needs to be printed and store it in ‘n’
- 2.initialize p0,p1,p2 to 1.
- 3.to generate pn or the n’th term, sum up p0 and p1 and store the resultant in pn.
- 4. While pn does not exceed n , the following steps need to be repeated:
- Assign 0 to ctr
- Check for the factors of pn and store the count of factors in ctr
- If ctr is equal to 2 then display pn.
- p0 is assigned value of p1
- p1 is assigned value of p2
- p2 is assigned value of pn
- pn is assigned the value of p0+p1
- 5.Stop
The following is the code written in C++ language to print the Prime Padovan Sequence when the number limit is provided by the user.
PROGRAM:
#include<iostream>
using namespace std;
int main()
{
int n;//number till which the series need to be printed
int i;//loop counter
int ctr;//count of factors of a number
cout<<"enter a number:";
cin>>n;
int p0=1;//first term of series
int p1=1;//second term of the series
int p2=1;//third term of the series
int pn=p0+p1;//current term of the series
while(pn<=n)
{
ctr=0;
for(i=1;i<=pn;i++)
{
if(pn%i==0)
ctr++;
}
if(ctr==2)
cout<<"\t"<<pn;
p0=p1;
p1=p2;
p2=pn;
pn=p0+p1;
}
return 0;
}
DRY RUN:
The dry run is very similar to the one in Padovan Sequence. Additionally, the value of "pn" is checked to see if it is prime or not. For more details about the dry run of Padovan Sequence , Click Here
OUTPUT:
enter a number:7
2 2 3 5 7