CURRENCY CONVERTER GUI USING JAVA AWT
AMRUHA AHMED
29th May,2023.
This article will help you to create a Currency Converter GUI using Java AWT.
Java AWT (Abstract Window Toolkit) is an API that aids in creation of window-based applications in Java Language.
Based on the current currency of a given amount, the task at hand is to convert it into its equivalent in another currency.
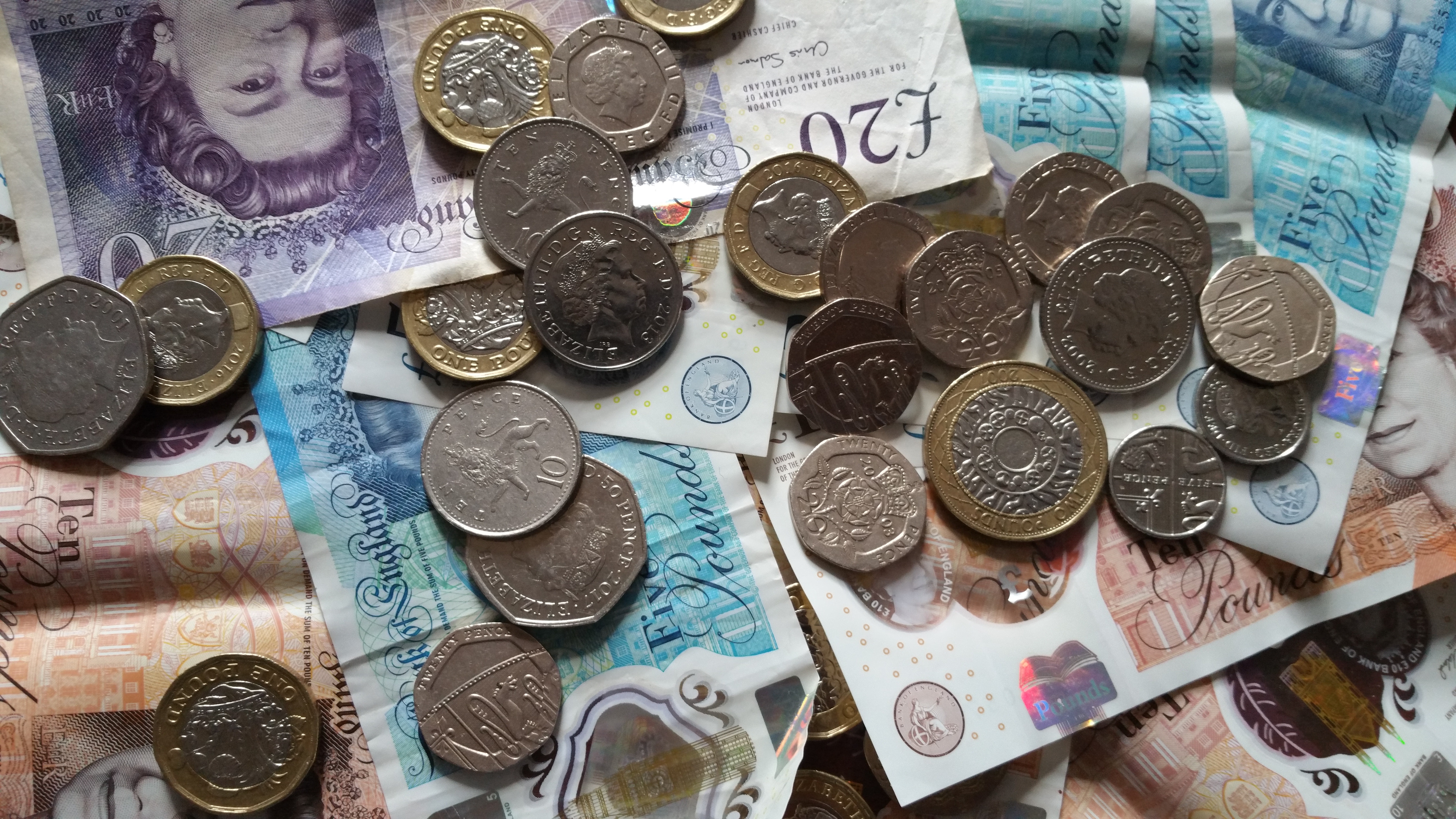
COMPONENTS OF THE INTERFACE:
- 1. Frame: to contain the other components of the interface.It is named as f.
-
2. Labels: to display a single line of text. They help in understanding the interface. Here, 5 labels are used:
- title: to display the heading
- l1: to display “Enter the amount”
- l2: to display “Select the current currency”
- l3: to display “Select the final currency “
- last: to display the amount converted from the current currency to the desired currency.
- 3.TextField: To accept the amount. It is named tf.
- 4.Choice : To display a menu of choices . In this program, two choices are used:
- c1: To show the menu of current currency of the amount entered
- c2: To show the menu of desired currency of the amount entered.
- 5.Button: When this button is clicked, the actionPerformed method is invoked that interprets all the values inputted and sets the text of the “last” label. It is named “b”.
DELVING DEEPER:
3 important parameters are required for making the Currency Converter:
- 1.Amount: It is the sum of money that needs to be converted
- 2.Given/Current Currency : It is the currency in which the amount is currently in.
- 3.Desired/Final Currency:It is the currency to which the amount needs to be converted to.
In this program, given currency is represented as curr1 and desired currency by curr2.
In this program, I have considered six different currencies:
- 1.Indian Rupee
- 2. United States Dollar
- 3.Euro
- 4.Pound Sterling
- 5.Chinese Yuan
- 6.Japanese Yen
These are stored in a String array named currency.
By a hunch, you may use the concept of if -else -if ladder for the conversions but there is a faster, more efficient way for converting the amount as you will observe.
If the if-else-if ladder is used then at least 35 conditions will be required for making the converter.
But, if we convert the amount in the given currency to an intermediate currency, then convert it to the desired currency, we can skip the hassle of writing a lengthy code.
Here, I am considering the intermediate currency to be Indian Rupees.
The conversion rates from the given currency to INR are stored in the given_to_rupee array.
I am also storing the conversion rates from INR to the desired currency in the rupee_to_final array.
Note: These conversion rates should be written in the same order as the currency array.
Whenever the user selects the current and desired currencies, their indexes in the currency array are fetched and stored in c1 and c2 respectively.
Now, using the conversion rate given at index c1 in the given_to_rupee array, “amount” is converted into INR . The result of this operation is stored in the current_to_rupee variable.
Using the conversion rate at index c2 in the rupee_to_final array, the “current_to_rupee” amount is converted to the desired currency. This final answer is stored in the rupee_to_desired variable.
This entire logic resides in the converter method.
PACKAGES TO BE IMPORTED:
- 1.java.awt package: for using the AWT Components
- 2.java.awt.event package: for dealing with the various events given by AWT components.
FUNCTIONS REQUIRED:
Firstly, a class named currencyconverter needs to be created that implements the ActionListener interface. The ActionListener interface is notified whenever the button is clicked.The functions used are:
- 1.converter( ): It contains the main logic of currency conversion as explained above.
- 2.actionPerformed():It is invoked when the button is clicked.It does the following:
- (i)It fetches the amount and the choices entered by the user
- (ii)Converts amount to double
- (iii)Passes these quantities as parameters to the converter method and stores the returned value in final_amt
- (iv)Sets the text of “last” label as final_amt
- 3.addComponents() : this adds the various components such as Button, Labels and TextField into the container.
- 4.boundSetter():this sets the bounds of the various components
- 5.fontSetter():this sets the font style,weight and size of all the text contained in the components.
- 6.currencyconverter():it initializes all the components of the interface and invokes the methods accordingly.
- 7. main(): it creates an object of the currencyconverter class.
PROGRAM:
The following program demonstrates how a currency converter GUI can be made using Java AWT:
import java.awt.*;
import java.awt.event.*;
class currencyconverter implements ActionListener
{
Frame f;
Button b;
Label title,l1,l2,l3,last;
TextField tf;
Choice c1,c2;
double converter(String curr1,String curr2,double amount)//converts amount from one currrency to another
{//curr1 is current/given currency, curr2 is the desired currency, "amount" is the amount to be converted
if(curr1.equals(curr2))// in case the current and desired currencies are the same
return amount;
String currency[]={"","Indian Rupee","United States Dollar","Euro","Pound Sterling","Chinese Yuan","Japanese Yen"};//array to store names of currencies
double given_to_rupee[]={0,1,82.60,88.52,101.88,11.69,0.59};//array to store conversion rates from given currency to INR
double rupee_to_final[]={0,1,0.012,0.011,0.0098,0.086,1.70};//array to store conversion rates from INR to desired currency
int c1=0;// stores index of given currency in currency array
int c2=0;// stores index of desired currency in currency array
for(int i=1;i<=6;i++)//loop to fetch indexes of given and desired currencies
{
if(curr1.equals(currency[i]))
c1=i;
if(curr2.equals(currency[i]))
c2=i;
}
double current_to_rupee=amount*given_to_rupee[c1];//converting amount in given currency to INR
double rupee_to_desired=current_to_rupee*rupee_to_final[c2];//converting current_to_rupee to desired currency
return(rupee_to_desired);
}
currencyconverter()
{//initializing all the components
f=new Frame("Currency Conversion");
title=new Label("Currency Converter GUI using Java AWT");
l1=new Label("Enter the amount:");
l2=new Label("Select the current currency :");
l3=new Label("Select the final currency :");
last=new Label();
b=new Button("Submit");
tf=new TextField();
c1=new Choice();
c2=new Choice();
//adding options in Choice
c1.add("Indian Rupee");
c1.add("United States Dollar");
c1.add("Euro");
c1.add("Pound Sterling");
c1.add("Chinese Yuan");
c1.add("Japanese Yen");
//adding options in Choice
c2.add("Indian Rupee");
c2.add("United States Dollar");
c2.add("Euro");
c2.add("Pound Sterling");
c2.add("Chinese Yuan");
c2.add("Japanese Yen");
b.addActionListener(this);//adding action listener to button
f.setVisible(true);//setting Visibility of Frame
f.setLayout(null);//setting Layout of Frame
// calling appropriate functions
boundSetter();
fontSetter();
addComponents();
}
void addComponents()
{//to add Components to the Frame
f.add(l1);
f.add(l2);
f.add(l3);
f.add(last);
f.add(title);
f.add(tf);
f.add(b);
f.add(c1);
f.add(c2);
f.add(b);
}
void fontSetter()
{//setting font style and size of the various components
title.setFont(new Font("Arial",Font.BOLD,35));
l1.setFont(new Font("Arial",Font.PLAIN,22));
l2.setFont(new Font("Arial",Font.PLAIN,22));
l3.setFont(new Font("Arial",Font.PLAIN,22));
tf.setFont(new Font("Arial",Font.BOLD,25));
c1.setFont(new Font("Arial",Font.PLAIN,22));
c2.setFont(new Font("Arial",Font.PLAIN,22));
b.setFont(new Font("Arial",Font.BOLD,25));
last.setFont(new Font("Arial",Font.PLAIN,22));
f.setBackground(Color.YELLOW);
b.setBackground(Color.PINK);
}
void boundSetter()
{//setting bounds of the various components
f.setSize(800,800);
title.setBounds(50,50,700,50);
l1.setBounds(50,150,300,50);
tf.setBounds(410,150,300,30);
l2.setBounds(50,250,300,50);
l3.setBounds(400,250,350,50);
c1.setBounds(50,350,250,200);
c2.setBounds(450,350,250,200);
last.setBounds(100,550,600,25);
b.setBounds(350,450,100,50);
}
public void actionPerformed(ActionEvent e)
{//executed when button is clicked
String amttext=tf.getText();//fetching text from TextField
double amount=Double.valueOf(amttext);//converting amttext to double
String currency1=c1.getSelectedItem();//get the option selected by user from 1st choice box
String currency2=c2.getSelectedItem();//get the option selected by user from 2nd choice box
double final_amt=converter(currency1,currency2,amount);//passing the required parameters to converter function
last.setText(amount+" of "+currency1+" in "+currency2+" is "+final_amt);//setting text of "last" label as the final amount
}
void main()
{//creating an object of current class
currencyconverter ob=new currencyconverter();
}
}
OUTPUT: