DISPLAYING AN ANIMATED CROSS PATTERN USING THREADS IN C LANGUAGE
AMRUHA AHMED
16th May,2023.
This article will help you to create an animated cross pattern using threads in C language. The cross pattern can be generated by using certain nested loops and the animation is achieved through the usage of threads.
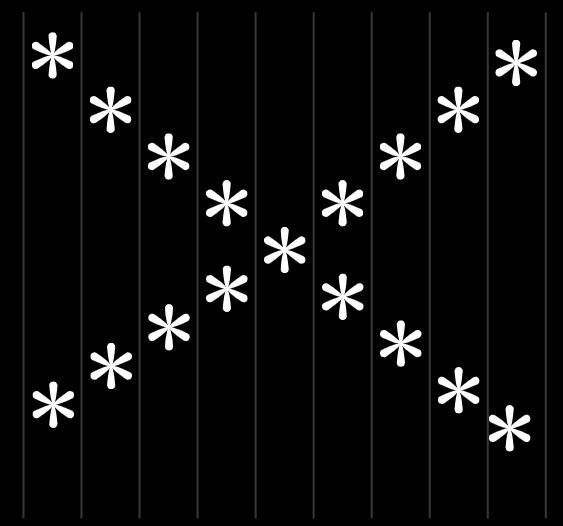
DELVE DEEPER INTO THE PATTERN:
- The Cross Pattern “X” requires the usage of nested loops to print the “*” as shown in the picture.
- It also needs two separate variables to keep a count of the spaces printed on the screen.
- The sleep method of the threads needs to be inserted at appropriate intervals to ensure the animated effect on the pattern.
BREAK UP OF THE UPPER HALF OF THE PATTERN:
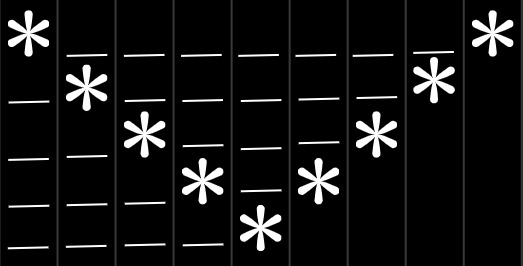
- 1.Five rows should be printed in the upper half of the pattern.
- 2.The dashes are an indication of the spaces required . The number of dashes outside the pattern are stored in variable "m". As observed, the number of dashes outside the pattern or "m" keeps increasing by 1 after each iteration i.e after printing the first “*” in each row. The number of spaces as indicated by “m” are printed in the current row.Hence before starting the loop,”m” is initialized to 0.
NOTE:this number “m” keeps varying according to the number of rows demanded by the question. - 3.Now, the first “*” is printed in the current row.
-
4.The number of dashes located in the middle of the pattern are stored in the variable “n”.As seen for the diagram, “n” is decreasing by 2 after printing the first “*” in each row. The number of spaces as indicated by “n” are printed in the current row. Hence, before starting the loop, “n” is initialized to 7.
NOTE:this number “n” keeps varying according to the number of rows demanded by the question. - 5.In case the value of the outer loop counter is equal to 5,the rest of the loop gets bypassed,since, a single “*” should be present in the 5th row.
- 6.If the outer loop counter is not equal to 5, the next “*” needs to be printed in the current row on the screen .
- 7.Now,the sleep function of the thread is introduced so that the lag between each iteration of the outer loop can give an animated effect to the pattern.
BREAK UP OF THE LOWER HALF OF THE PATTERN:
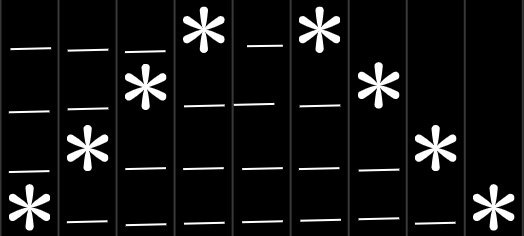
- 1.The lower half of the pattern has 4 rows , with each row having two “*” separated by a space
- 2.The number of dashes outside the pattern are stored in variable "m". As observed, the number of dashes outside the pattern or "m" keeps decreasing by 1 after each iteration i.e after printing the first “*” in each row. The number of spaces as indicated by “m” are printed in the current row.Hence before starting the loop,”m” is initialized to 3.
NOTE:this number “m” keeps varying according to the number of rows demanded by the question. - 3.Now, the first “*” is printed in the current row.
-
4.The number of dashes located in the middle of the pattern are stored in the variable “n”.As seen for the diagram, “n” is increasing by 2 after printing the first “*” in each row. The number of spaces as indicated by “n” are printed in the current row. Hence, before starting the loop, “n” is initialized to 1.
NOTE:this number “n” keeps varying according to the number of rows demanded by the question - 5.The second “*” is printed on the screen.
- 6.Now,the sleep function of the thread is introduced so that the lag between each iteration of the outer loop can give an animated effect to the pattern.
One thing to be kept in mind is that, all these instructions of pattern creation are present in the runner method of the program;these instructions are exceuted by a thread created in the main method.
FUNCTIONS REQUIRED:
- main()- main()-to create a thread
- *runner()-contains the set of instructions required to generate the animated pattern.It is executed by the thread created in the main method. After the desired instructions are executed, it terminates the thread.
PROGRAM:
The following is the code written in C to print the desired pattern on the screen and add animations to it by using the concept of threads:
#include<stdio.h>
#include<stdlib.h>
#include<pthread.h>
#include<unistd.h>
void *runner();
void main()
{
pthread_t tid;//thread id
int ret;//to store a return value
ret=pthread_create(&tid,NULL,runner,0);//creating a thread
if(ret==-1)
{
printf("\n Thread has not been created");
exit(1);
}
pthread_join(tid,0);
}
void *runner()
{
int i,j,m,n;// i and j are loop counters
// m and n are used as counters for spacing
m=0;
n=7;
for(i=1;i<=5;i++)
{//to generate upper half of the pattern
for(j=1;j<=m;j++)
{
printf(" ");
}
printf("* ");
for(j=1;j<=n;j++)
printf(" ");
m+=1;
n-=2;
if(i==5)
continue;
printf("* ");
sleep(2);
printf("\n");
}
n=1;
m=3;
printf("\n");
for(i=1;i<=4;i++)
{ //to generate lower half of the pattern
for(j=1;j<=m;j++)
printf(" ");
printf("* ");
for(j=1;j<=n;j++)
printf(" ");
printf("* ");
sleep(2);
m-=1;
n+=2;
printf("\n");
}
pthread_exit(0);
}
OUTPUT: