ANIMATED SPLIT PYRAMID PATTERN IN C LANGUAGE
AMRUHA AHMED
9th October,2023.
This article will aid you in the creation of an animated split pyramid pattern in C language.This pattern is generated using a combination of nested loops and the animation is provided by threads.
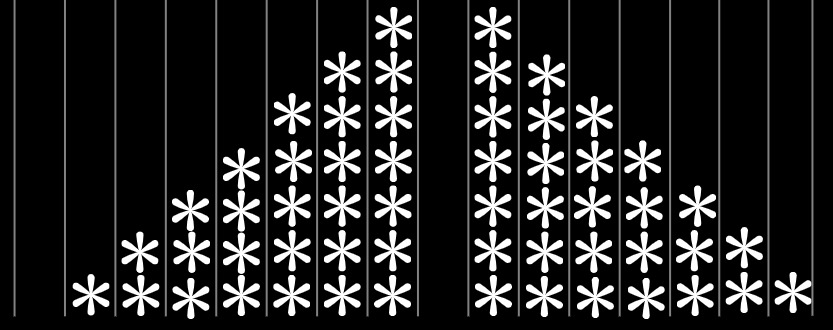
DELVING DEEPER:
- 1.This pattern requires the usage of nested loops to print the asterisks in the appropriate manner
- 2.One separate variable is required to check the number of spaces printed on the screen before the asterisks are printed
- 3.The sleep method of threads needs to be inserted at suitable intervals to impart an animated look and feel to the split pyramid
BREAK-UP OF THE PATTERN:
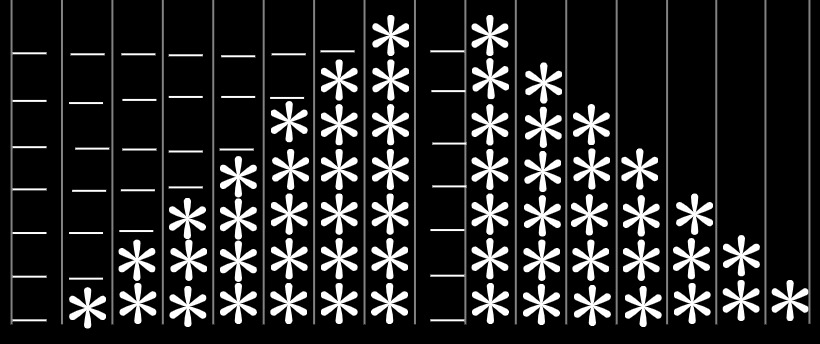
- 1.A total of 7 rows need to be printed in the pattern.
- 2.On each side of the split, the asterisks keep increasing by 1 after each iteration.
- 3.The dashes are an indication of spaces required. Before the outer loop begins, the space variable is initialized to 7, that is, the number of rows of asterisks to be printed on the screen.As observed from the above pattern, the spaces keep decreasing by 1 after each iteration.
- 4.The set of asterisks on the left side of the split are printed on the screen depending on the value of the outer loop counter.
- 5.Sleep method is introduced to provide a lag after execution of the above step
- 6.A space is printed on the screen to impart the split look to the pyramid.
- 7.The number of asterisks to be printed on the right side of the split are determined by the value of the outer loop counter.
- Sleep method is introduced again to provide a lag after execution of the above step
FUNCTIONS REQUIRED:
- 1. main()- used for thread creation
- 2.*runner()-Contains the instructions required to generate the desired pattern. This method is executed only if the thread has been created successfully in the main method.
PROGRAM:
#include<stdio.h>
#include<stdlib.h>
#include<pthread.h>
#include<unistd.h>
void *runner();
void main()
{
pthread_t tid;//thread id
int ret;//storing the return value
ret=pthread_create(&tid,NULL,runner,0);//thread creation
if(ret==-1)
{
printf("\n thread is not created ");
exit(1);
}
pthread_join(tid,0);
}
void *runner()
{
int s=7;//number of spaces to be printed
int i,j;//loop counters
for(i=1;i<=7;i++)
{
for(j=1;j<=s;j++)
{
printf(" ");
}
for(j=1;j<=i;j++)
{
printf("* ");
}
sleep(1);
printf(" ");
for(j=1;j<=i;j++)
{
printf("* ");
}
sleep(1);
printf("\n");
s--;
}
pthread_exit(0);
}
OUTPUT: