ANIMATED HOURGLASS PATTERN IN C LANGUAGE
AMRUHA AHMED
17th June,2023.
This article will help you create an animated hourglass pattern in C language.This pattern can be generated using nested loops and the animated effect is provided by the usage of threads.
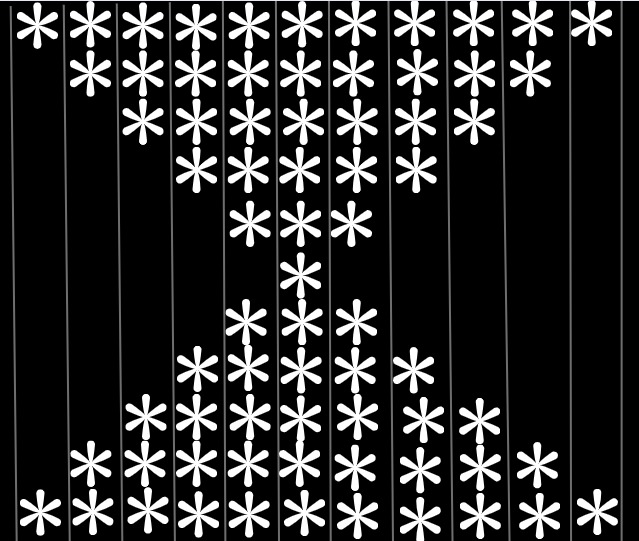
DELVING DEEPER:
- 1.The hourglass pattern requires the usage of nested loops to print “*” in the appropriate manner as shown
- 2.One separate variable is required to keep in check the number of asterisks printed on the screen.
- 3.Another variable is required to keep track of the number of spaces printed on the screen.
- 4.The sleep method of threads also needs to be inserted at appropriate intervals to ensure the animated effect on the hourglass pattern.
BREAK-UP OF THE UPPER HALF OF THE PATTERN:
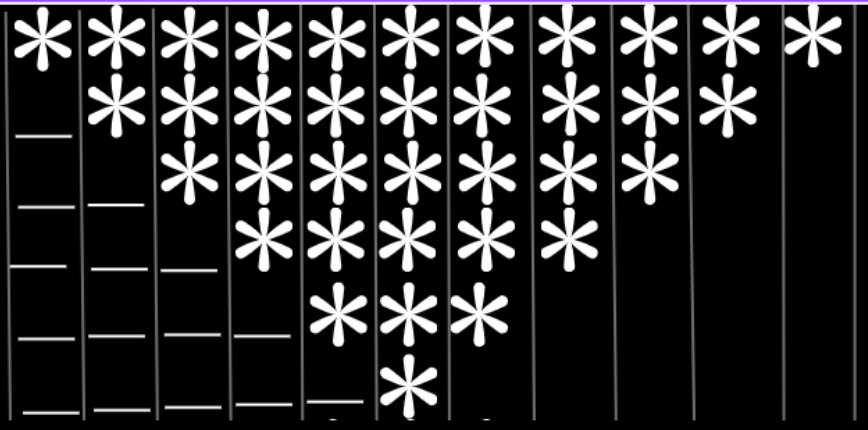
- 1. 6 rows need to be printed in the upper half of the pattern
- 2. The dashes are an indication of the spaces required. The number of dashes outside the pattern are stored in variable “s”.Before starting the outer loop, “s” is initialized to 0. As observed from the image given above, the number of spaces keeps on increasing by 1 after each iteration. These spaces indicated by “s” are printed in the current row.
NOTE: Value of “s” depends upon the number of rows to be printed on the screen - 3.The number of asterisks to be printed in the current row are denoted by “m”. Before starting the loop, “m” is initialized to 11.As inferred from the image above, the number of asterisks keeps on decreasing by 2 after each iteration.
NOTE: Value of “m” depends upon the number of rows to be printed on the screen - 4.Within the outer loop, the sleep method of thread is introduced so that the lag between each iteration can give an animated effect to the pattern.
BREAK-UP OF THE LOWER HALF OF THE PATTERN:
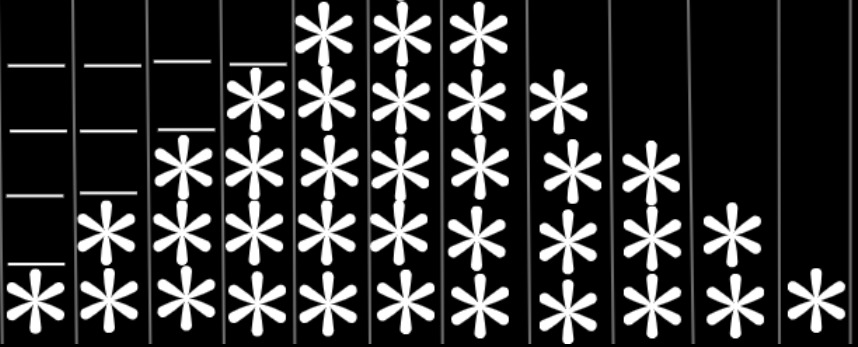
- 1.The lower half of the pattern has 5 rows.
- 2. The dashes are an indication of the spaces required. The number of dashes outside the pattern are stored in variable “s”.Before starting the outer loop, “s” is initialized to 4. As observed from the image given above, the number of spaces keeps on decreasing by 1 after each iteration. These spaces indicated by “s” are printed in the current row.
NOTE: Value of “s” depends upon the number of rows to be printed on the screen - 3.The number of asterisks to be printed in the current row are denoted by “m”. Before starting the outer loop, “m” is initialized to 3.As inferred from the image above, the number of asterisks keeps on increasing by 2 after each iteration.
NOTE: Value of “m” depends upon the number of rows to be printed on the screen - 4.Within the outer loop, the sleep method of thread is introduced so that the lag between each iteration can give an animated effect to the pattern.
All these instructions of pattern creation are present in the runner method and get executed when a thread is created in the main method.
FUNCTIONS REQUIRED:
- 1.main(): used for creation of thread
- 2.*runner (): It contains instructions required to generate the pattern. This method is executed when a thread is created in the main method. After executing these statements, it terminates the threads.
PROGRAM:
The following program in C language prints the desired pattern on the screen and adds animations to it:
#include<stdio.h>
#include<stdlib.h>
#include<pthread.h>
#include<unistd.h>
void *runner();
void main()
{
pthread_t tid;//thread id
int ret;//to store a return value
ret=pthread_create(&tid,NULL,runner,0);//creating a thread
if(ret==-1)
{
printf("\n Thread has not been created");
exit(1);
}
pthread_join(tid,0);
}
void *runner()
{
int m=11;//number of asterisks to be printed
int s=0;// number of spaces
int i,j;//i-outer loop counter, j-inner loop counter
//upper half of the pattern
for(i=1;i<=6;i++)
{
for(j=1;j<=s;j++)
printf(" ");
for(j=1;j<=m;j++)
printf("* ");
sleep(1);
s++;
m-=2;
printf("\n");
}
m=3;
s=4;
//lower half of the pattern
for(i=1;i<=5;i++)
{
for(j=1;j<=s;j++)
printf(" ");
for(j=1;j<=m;j++)
printf("* ");
sleep(1);
s--;
m+=2;
printf("\n");
}
pthread_exit(0);
}
OUTPUT: