AFFINE CIPHER IN JAVA
AMRUHA AHMED
3rd April,2024.
Affine cipher is a simple encryption technique that follows monoalphabetic substitution. This cipher converts plaintext to ciphertext by making use of the numerical equivalent of alphabets . The formula used for encryption is:
ciphertext=((a*plaintext)+b)%26
Where the plaintext refers to numerical equivalent of the plaintext alphabet(such as 0 for A, 1 for B and so on ). Ciphertext refers to the numerical equivalent of the ciphertext alphabet. 'a' is the multiplier and 'b' is the additive shift value. Both 'a' and 'b' serve as keys to this encryption technique.
The value obtained from ciphertext will be converted to the equivalent character.
Though relatively easy to understand and implement, it is shadowed by more secure encryption techniques.
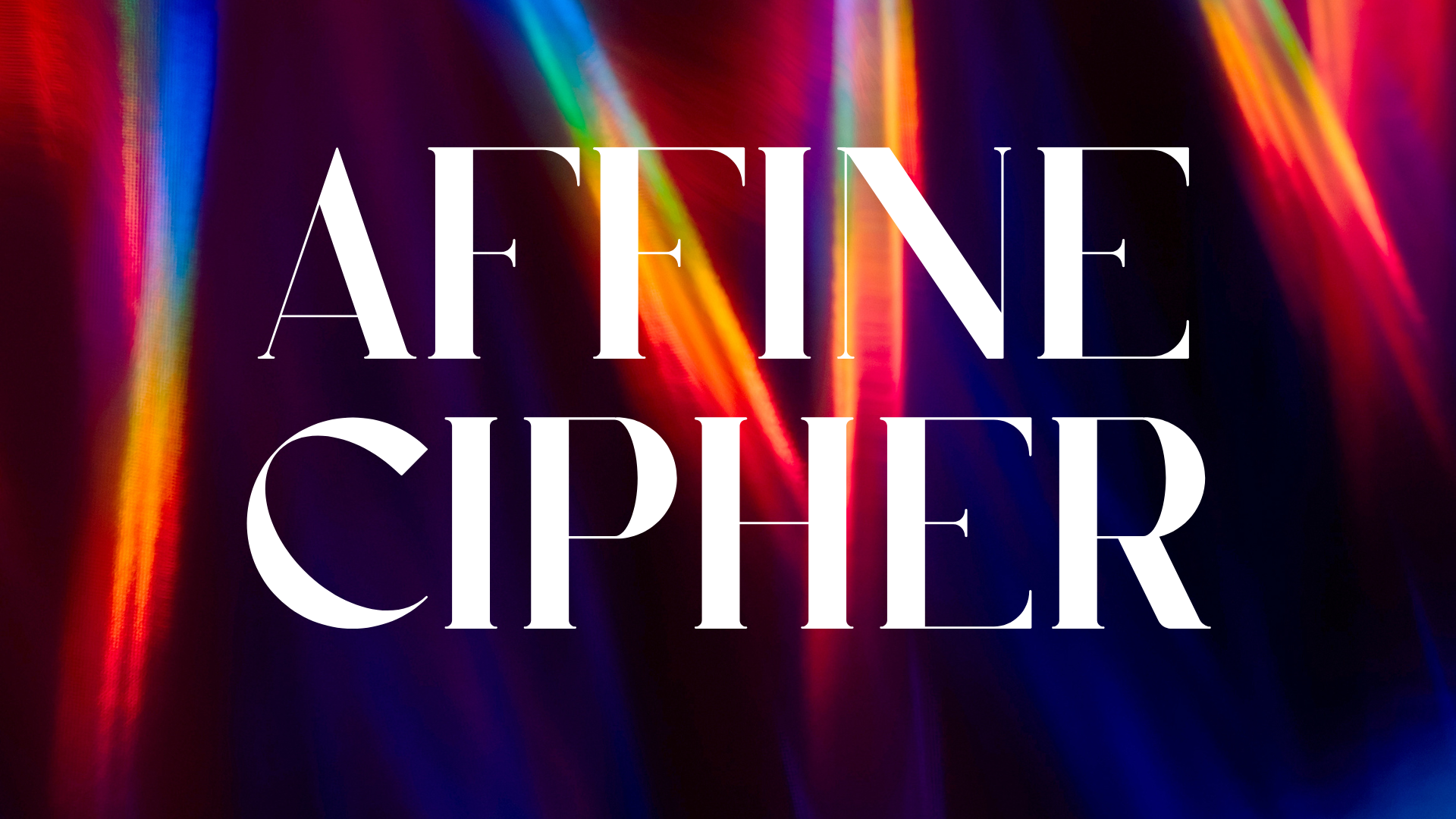
ARRAYS USED IN THE AFFINE CIPHER PROGRAM:
- 1.alpha-array of alphabets from A to Z
- 2.number-array of numerical equivalent of alphabets
VARIABLES IN AFFINE CIPHER PROGRAM:
- 1.a - multiplier
- 2.b - additive shift value
- 3.plaintext - to store the plaintext given by the user
- 4.ciphertext - to store the ciphertext generated after computation
- 5.i - loop counter
- 6.j - loop counter
- 7.start - starting character in alpha array
- 8.cc - storing ciphertext of current letter
- 9.pos - stores numerical equivalent of character
- 10.c - to store the current character of the plaintext
ALGORITHM FOR AFFINE CIPHER:
- 1.Start
- 2.Accept the values of 'a' , 'b' and the plaintext from the user
- 3.Convert the plaintext to uppercase
- 4.Initialize the number array with the numerical equivalent of each alphabet such as 0-> A, 1->B ….. 25->Z and store the corresponding alphabets in the alpha array
- 5.Until j is less than the length of plaintext, repeat the steps from 6 to 10
- 6.Get the current character of plaintext and store in c
- 7.Initialize pos to 0
- 8.Find numerical equivalent of character 'c' and store it in pos
- 9.Calculate the numerical equivalent of cipher text using the formula ((a*pos)+b)%26 and store it in cc
- 10.Include the encrypted character into the ciphertext string by concatenating alpha[cc] (alphabet corresponding to cc numerical value) to ciphertext
- 11.Display ciphertext
- 12.Stop
PROGRAM:
import java.util.*;
class affinecipher
{
Scanner ob=new Scanner(System.in);
void main()
{
int a,b;// a- multiplier, b-additive shift value
String plaintext,ciphertext="";//to store plaintext and cipher text respectively
int i,j;//i and j are loop counters
int cc,pos;//cc- storing ciphertext of current letter, pos-stores numerical equivalent of character
char start='A';//starting character in alpha array
int number[]=new int[26];//array of numerical equivalent of alphabets
char alpha[]=new char[26];//array of alphabets from A to Z
System.out.print("Enter the multiplier 'a':");
a=ob.nextInt();
System.out.print("Enter the additive shift 'b':");
b=ob.nextInt();
System.out.print("Enter the plaintext:");
plaintext=ob.next();
plaintext=plaintext.toUpperCase();
for(i=0;i<26;i++)
{//storing current alphabet in alpha and its numerical equivalent in number
number[i]=i;
alpha[i]=start;
start++;
}
for(j=0;j<plaintext.length();j++)
{
char c=plaintext.charAt(j); //getting the current character of plaintext
pos=0;
for(i=0;i<alpha.length;i++)
{//finding numerical equivalent of 'c'
if(c==alpha[i])
{
pos=i;
break;
}
}
cc=((a*pos)+b)%26;//calculating ciphertext of alphabet 'c'
ciphertext=ciphertext+""+alpha[cc];//updating the ciphertext string
}
System.out.println("The cipher text is "+ciphertext);
}
}
DRY RUN:
Suppose if the user enters plaintext as "SECRET", a as 5 and b as 8 , then the computation is carried out as follows: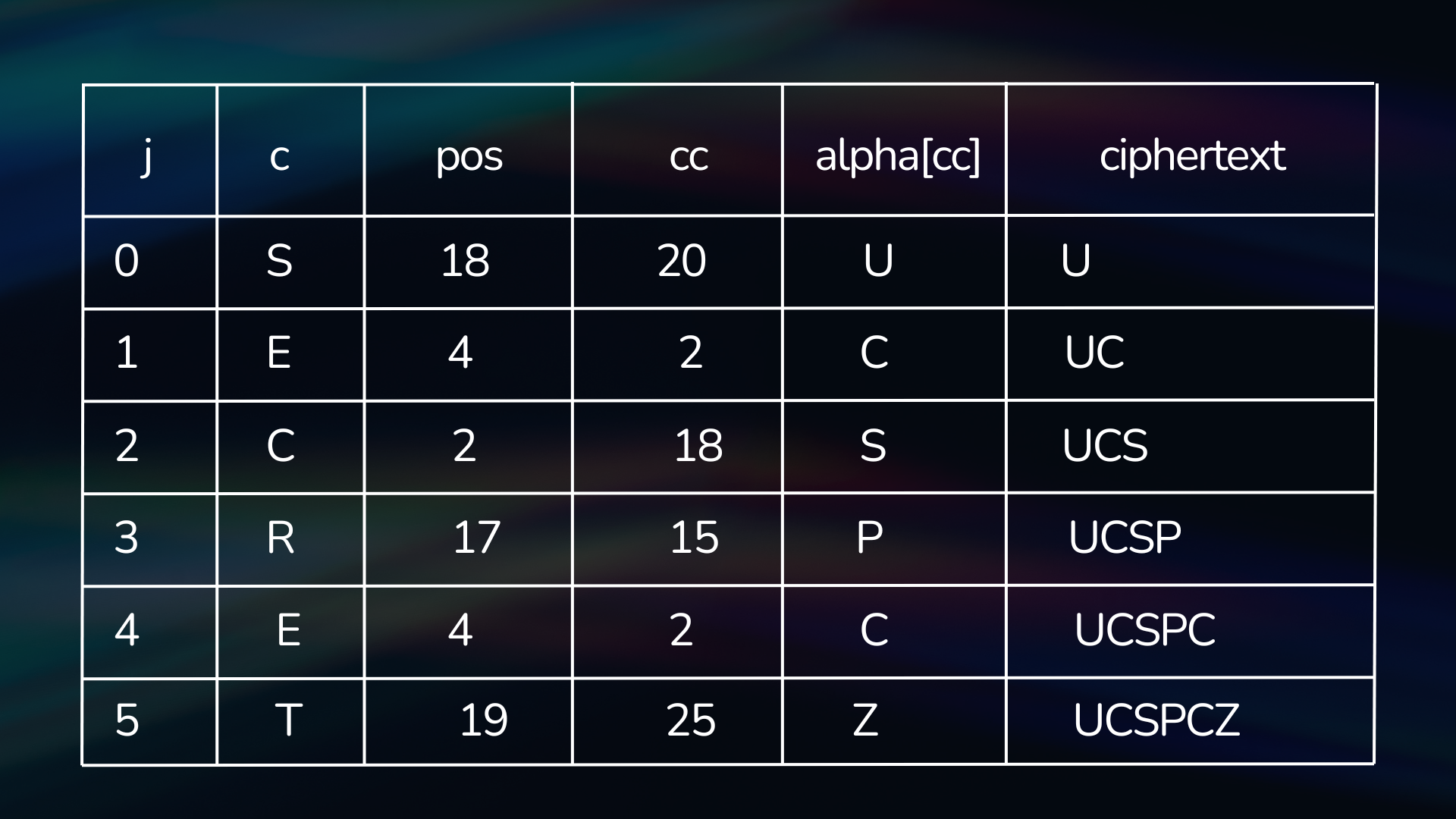
The final cipher text obtained is UCSPCZ
OUTPUT:
Enter the multiplier 'a':5
Enter the additive shift 'b':8
Enter the plaintext:SECRET
The cipher text is UCSPCZ